Uma Trilha de Auditoria (também chamada de Log de Auditoria) é uma das maneiras mais eficazes de rastrear as ações dos usuários, fornecendo evidências de uma sequência de atividades que afetam informações, processos etc.
Usando o Entity Framework Core, podemos facilmente auditar as alterações, sobrescrevendo o método SaveChanges ().
Vamos ao que interessa
Defina a classe context e as classes de entidade que conformam seu modelo:
Agora, devemos definir uma classe context auditável e o modelo AuditTrail:
Com os comandos de migração EF, criamos nossa base de dados e suas tabelas:
dotnet ef migrations add InitialCreate --context BloggingContext
dotnet ef database update --context BloggingContext
Vamos aos testes
Adicionaremos dados a nossa tabela Blog:
INSERT INTO [Blogging].[dbo].[Blogs] (Url)
VALUES ('blog.victorleonardo.com');
Agora, devemos efetuar uma atualização ao registro que acabamos de inserir, utilizando nossa classe context auditável:
Voilà:
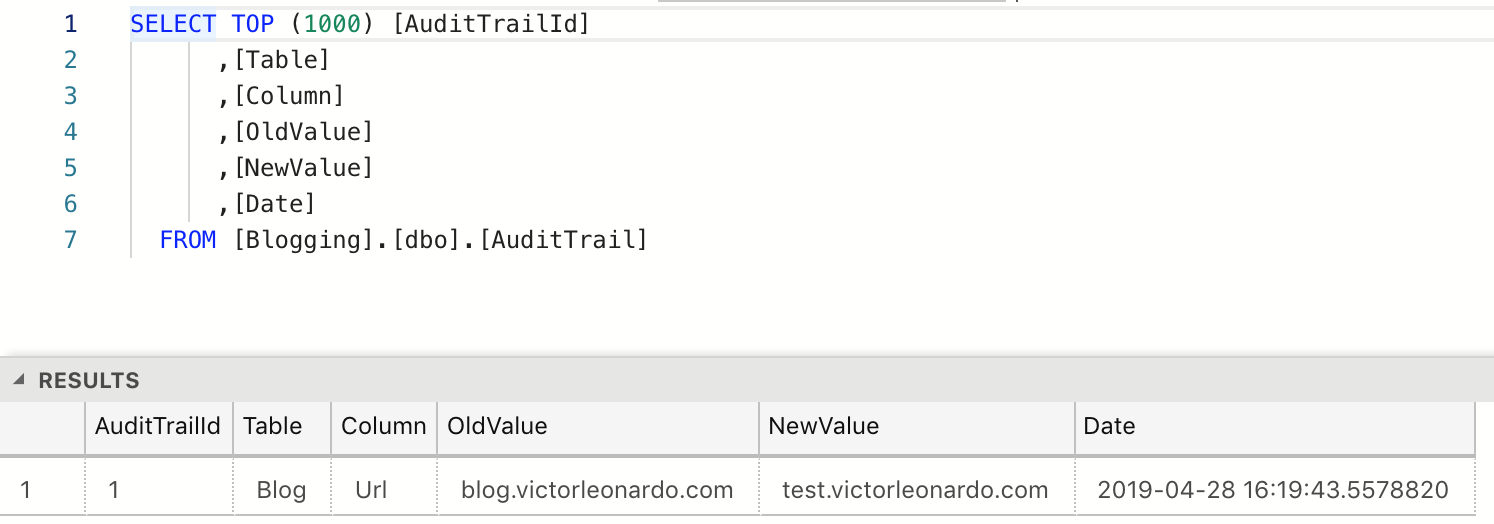